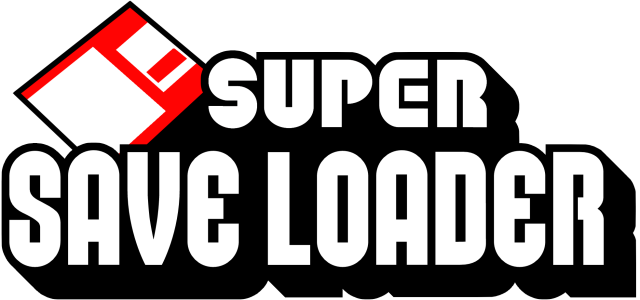
Super Save Loader Documentation - v2.0
Super Save Loader Documentation
Super Save Loader Documentation - v2.0
To import Super Save Loader into your project, either drag in the .unitypackage file, or get it from the asset store window.
The rest of the files are required.
For the following examples on saving, I will be using the JSON serializer. To use the XML serializer, just replace "JSON" with "XML" in your code!
This line of code tells Super Save Loader to save an object named "myObject" as "savedobject.save" without using encryption:
SSL.SaveJSON(myObject, "savedobject.save", false);
This line of code is a shorthand for the above one, saving without encryption:
SSL.SaveJSON(myObject, "savedobject.save");
There are two ways to load objects, either as a new object, or as an overwrite to old ones. Code to overwrite an object named "myObject" from a file named "savedobject.json" would look like this:
SSL.LoadJSON(ref myObject, "savedobject.json", false);
While the code for creating a new object would look like this:
MyObjectType myObject = SSL.LoadJSON<MyObjectType>("savedobject.json", false);
The boolean at the end controls whether encryption is used or not, and a shorthand like the one above for saving works for loading, too.
To load data from other games, you have to provide the Developer Name and the Product Name. These will be the names of the folders within the appdata folder or equivalent. For example, if you were to download my game "Hotel Paradise", my Developer Name is "KaiClavier" and my Product Name is "HotelParadise", so the folder route would be [appdata folder/KaiClavier/HotelParadise/]
. You can find the developer and product names of a game this way.
The functions to load are the same, just with two extra strings for the developer name and product name, respectively.
SSL.LoadJSON(ref myObject, "savedobject.json", "KaiClavier", "HotelParadise", false);
If you're loading from another game on Android, you also need to provide the "bundle identifier". This can be left out if your project isn't on Android.
SSL.LoadJSON(ref myObject, "savedobject.json", "KaiClavier", "HotelParadise", "my.bundle.identifier", false);
You can still load obfuscated data from other games!
To serialize custom objects, you need to use attributes! Attributes (also called "sub properties") are those lines of text between brackets like [this]
. To use them, place them before a class/variable, either in the previous or same line. Also, if applicable, attributes can be stacked!
[System.Serializable] <= This attribute is required for it to be saved! You need to use this on custom classes!
public class MyObject{
int foo;
[System.NonSerialized] float bar; <= This attribute means "bar" will be excluded from being saved with MyObject
}
The following attributes are to make XML code look cleaner. You only need to use them if you're picky about files no one will see.
using System.Xml.Serialization; <= Put this at the top of your script to use these attributes.
[System.Serializable]
[XmlRoot("GameData")] <= Renames root class in the XML file to "Game"!
public class MyObject{ <= By default, "MyObject" would be the root class instead.
int foo;
[XmlAttribute("extra")] <= Changes the name of "bar" to "extra" in the XML file!
float bar;
}
You cannot use [XmlAttribute("myLabel")] on Lists, Arrays, Vector3s, or any other class that contains more than one variable. It won't work, and it'll return an error.
To set Product Name & Company Name for your game:
Go to [Edit > Project Settings > Player]
, and they're the top two things in the inspector!
To set the Bundle Identifier: (for mobile games)
Go to [Edit > Project Settings > Player]
, then click on Android or iOS, then look for "Bundle Identifier"!
Make sure the textures you want to save are read/write enabled. Textures may also save improperly if mipmapping is enabled or transparency is disabled.
LoadTXT() can be used on non-txt files to get them as a string! Try it out with JSON or XML files.
Files dont have to end with .json or .txt, you can give them a made-up extention like .save or .playerdata
If you include forward slashes in your filename ("mysubfolder/anotherfolder/file.txt"), your files can be sorted into folders within the persistent data folder!
Save an object as JSON.
Overwrite an object from a JSON file.
Create a new object from a JSON file.
Save an object as XML.
Overwrite an object from a XML file.
Create a new object from a XML file.
Save a string as a TXT file.
Return a TXT file as a string, or read any file as a string.
Save a Texture2D as a PNG. Be wary of quality.
Save a Texture2D as a JPEG. Can control JPEG compression levels with the quality integer, which goes from 0 to 100.
Load an image as a Texture2D. Function used doesn't actually matter, as long as its a PNG or a JPEG that's being loaded.
Returns true if a game's save data exists on this device.
Returns true if a specific save file exists on this device.
Coding and design by Kai Clavier (@KaiClavier)
Extra CSS help by Tak (@takorii)